Resources:
https://playwright.dev/docs/codegen
Installation:
npm init playwright@latest
During installation, you’ll be prompted with several options. Here’s an example of what I selected:
Initializing project in '.'
Do you want to use TypeScript or JavaScript? · JavaScript
Where to put your end-to-end tests? · tests
Add a GitHub Actions workflow? (y/N) · false
Install Playwright browsers (can be done manually via 'npx playwright install')? (Y/n) · true
Once installed, you can use the following commands from your project’s root directory:
npx playwright test
Runs the end-to-end tests.
npx playwright test --ui
Starts the interactive UI mode.
npx playwright test --project=chromium
Runs the tests only on Desktop Chrome.
npx playwright test example
Runs the tests in a specific file.
npx playwright test --debug
Runs the tests in debug mode.
npx playwright codegen
Auto generate tests with Codegen.
The installation also comes with an example folder, tests-examples
, which is really handy for getting a quick grasp of how to use this tool. Running the command npx playwright test example
will execute example test cases, and you’ll see something like 6/6 tests passing.
The command npx playwright codegen
opens up an empty Codegen browser, but you can also specify a URL, like this:
npx playwright codegen "http://study.369usa.com/demo.html"
After playing around in the Codegen browser, it generates a list of actions in clean JavaScript for me:
import { test, expect } from '@playwright/test';
test('test', async ({ page }) => {
await page.goto('http://study.369usa.com/demo.html');
await page.getByLabel('Number A:').click();
await page.getByLabel('Number A:').fill('1');
await page.getByLabel('Number B:').click();
await page.getByLabel('Number B:').fill('2');
await page.getByRole('button', { name: 'Add Numbers' }).click();
await expect(page.getByTestId('result')).toContainText('3');
});
A special mention for the assertion parts: at the top of the Playwright Inspector, you’ll find three buttons—Assert Visibility, Assert Text, and Assert Value—to help with adding assertions without the need of writing a single line of your own code.
Once the test is successfully generated, simply copy and paste it into a test file, name it xxxx.spec.js
, and run the command npx playwright test
. As expected, the single test I just created passed!
Additionally, by running:
npx playwright test --ui
You can even see the test steps play out one by one. You can control the steps, go back and forth, and interact with the simulated browser.
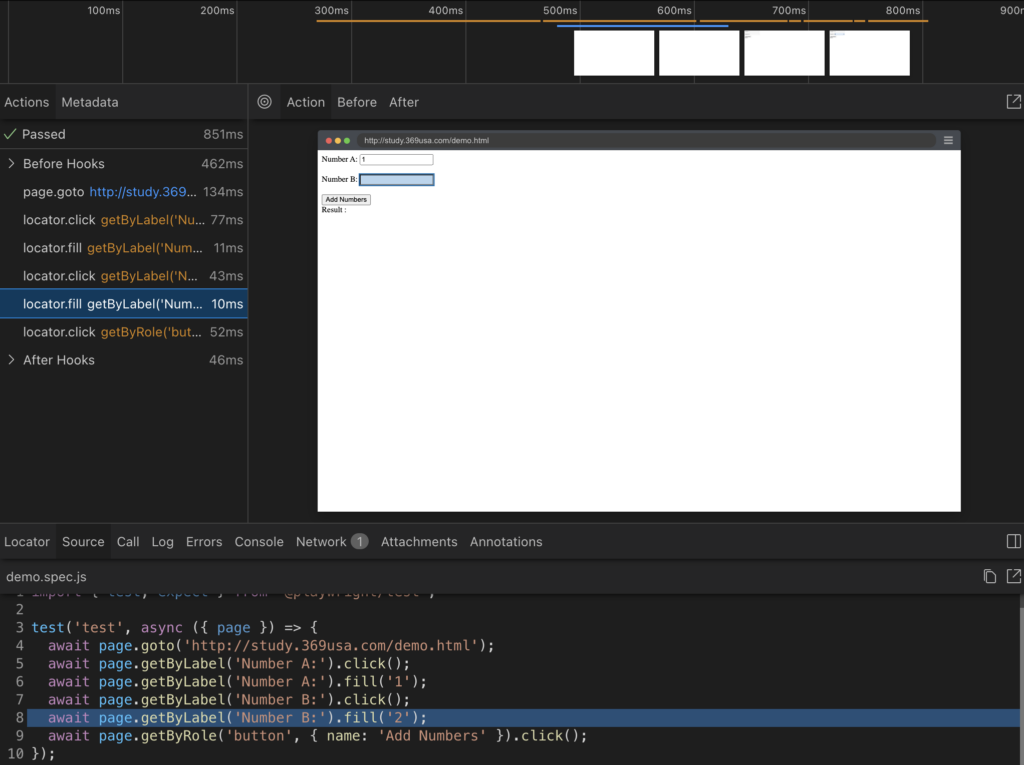
I don’t know about you, but I’ve definitely fallen in love with it!
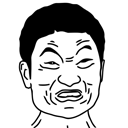